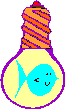 |
A Registry Class
A Registry Library
|
|
This represents a handy set of classes I built to ease the pain of dealing
with the Registry. In addition to a set of high-level classes, defined in
RegVars.h, there is a separate library, used by the RegVars module,
Registry.h. This includes the download link for the complete source for all four
files. The rest of this document describes the interface.
Using the Registry can be inconvenient. You have to learn all the Registry
APIs, learn how to create keys, and then discover that you have to create the
keys recursively. A real pain.
I created these classes and library to make it easy to do simple tasks with
the Registry. It requires that you defined several strings in the String Table
of the module that contains it, the most important one being
IDS_PROGRAM_ROOT "Software\\YourCompanyName\\YourProductName"
All accesses to the Registry via this library will be relative to this key.
For most simple cases, such as integers or strings, you can use the Registry
classes RegistryInt,
RegistryInt64
RegistryString,
RegistryGUID, RegistryWindowPlacement,
RegistryFont, and
RegistryDWordArray.
To use these, you simply declare a String Table entry with the desired access
key, which itself may contain \ characters for sub-paths.
For example, a simple value could stored using a simple string. First, create in
the STRINGTABLE a variable with the desired name.
IDS_REGISTRY_COUNT "Count"
and then declare a variable of the form
RegistryInt count(IDS_REGISTRY_COUNT);
and to load it you write
count.load(defaultvalue); // argument optional, 0 assumed
You can then access the value, which is of type int, by using
count.value
If you want to store the value in the Registry, set count.value to the
value you want to store, and do
count.store();
and the value is stored in the Registry. If the steps of the path do not
exist, they will be created.
The corresponding string type is RegistryString and the .value
member is a CString.
RegistryString s(IDS_REGISTRY_NAME);
s.load();; // defaults to ""
will load a string value from the Registry.
To use a structured value, you can just use the \
in the path name. For example,
IDS_REGISTRY_COLOR_RED "Color\Red"
IDS_REGISTTRY_COLOR_GREEN "Color\Green"
IDS_REGISTRY_COLOR_BLUE "Color\Blue"
To save the color information for the application, you could write something
like
void SaveColor(COLORREF c)
{
RegistryInt red(IDS_REGISTRY_COLOR_RED);
red.value = GetRValue(c);
red.store();
RegistryInt green(IDS_REGISTRY_COLOR_GREEN);
green.value = GetGValue(c);
green.store();
RegistryInt blue(IDS_REGISTRY_COLOR_BLUE);
blue.value = GetBValue(c);
blue.store();
}
The Registry Library underlies the Registry classes, and provides more
detailed control. I did not provide classes for some of the interfaces because
they are used infrequently enough that I didn't bother creating classes for
them.
The Registry Library allows for the setting and retrieving of string
values, integer values, 64-bit
integer values, GUIDs, font
descriptions, window placement
descriptions, and arrays of DWORD
values, as well as the ability to delete
keys and values, enumerating keys and values, and simply getting a handle to
a key for more detailed Registry API operations.
Like the Registry classes, these always work relative to
IDS_PROGRAM_ROOT
and automatically create steps of the path on setting operations.
Whenever a string ID is derived from the STRINGTABLE, I use the
notation %id% to indicate the
result obtained by doing LoadString(id). So if
IDS_PROGRAM_ROOT is "JMNCO\MyProgram" and
IDS_COLOR is "Color", then the string
%IDS_PROGRAM_ROOT%\%IDS_COLOR% represents the string
JMNCO\MyProgram\Color.
Compiling and Linking
You must compile and link in Registry.cpp to
get any of these facilities. You only need to include Registry.h
if you are using specific functions. RegVars.h
defines the RegistryInt and RegistryString variables, and does not
require Registry.h to be included in your
compilations, but you must also compile and link in RegVars.cpp
to get these classes.
This package requires you link with shlwapi.lib
to get a correct link.
Although to use this you only need to include this header file, you must link
with the Registry library, which the methods used by
these classes call to perform the actual Registry operations. If you include
this file in any of your project files, you must include RegVars.cpp
in your project or link with the compiled version RegVars.obj.
All registry variable types have the following method defined:
Deletes the registry variable. If this method returns FALSE, then
::GetLastError will return the reason for the failure.
RegistryInt::RegistryInt(UINT id, HKEY r = HKEY_CURRENT_USER)
|
Constructs a variable of type RegistryInt. This will save any
32-bit signed or unsigned value. Shorter values should be saved as this type.
The Registry value is stored as a REG_DWORD.
Parmeters:
UINT id
|
The String Table ID of the Registry key under
which the value will be stored or from which the value will be retrieved. |
HKEY r
|
The root key under which the key is specified.
It will be found under the key
r\%IDS_PROGRAM_ROOT%\%id%
|
BOOL RegistryInt::load(int def = 0);
|
Loads a value from the Registry. Provides for a default value. The .value
member of the variable will have either the value from the Registry or the
default value when this method returns. If it returns FALSE, use
::GetLastError to determine why it failed. If the value in the Registry is
not a REG_DWORD, the operation will fail with ERROR_INVALID_DATATYPE.
If the value is not found in the Registry, the return type will be TRUE.
In this case, ::GetLastError will return ERROR_FILE_NOT_FOUND. If
the return type is TRUE and ::GetLastError returns
ERROR_SUCCESS it means the value was actually read from the Registry.
int def
|
The value to be used if the key is not found in
the Registry. |
BOOL RegistryInt::store();
|
Stores the value to the Registry under the key specified for the variable.
The current contents of the .value member is what will be stored. If it
returns FALSE, use ::GetLastError to determine why it failed.
This is the .value member of the class.
RegistryInt64::RegistryInt64(UINT id, HKEY r = HKEY_CURRENT_USER)
|
Constructs a variable of type RegistryInt64. This will save any
32-bit signed or unsigned value. Shorter values should be saved as this type.
The Registry value is stored as a REG_BINARY.
Parmeters:
UINT id
|
The String Table ID of the Registry key under
which the value will be stored or from which the value will be retrieved. |
HKEY r
|
The root key under which the key is specified.
It will be found under the key
r\%IDS_PROGRAM_ROOT%\%id%
|
BOOL RegistryInt64::load(__int64 def = 0);
|
Loads a value from the Registry. Provides for a default value. The .value
member of the variable will have either the value from the Registry or the
default value when this method returns. If it returns FALSE, use
::GetLastError to determine why it failed. If the value in the Registry is
not a REG_BINARY the operation will fail with ERROR_INVALID_DATATYPE.
If the length of the value is not sizeof(__int64) the operation will fail
with ERROR_INVALID_DATA. If the value is not found in the Registry, the
return type will be TRUE. In this case, ::GetLastError will return
ERROR_FILE_NOT_FOUND. If the return type is TRUE and
::GetLastError returns ERROR_SUCCESS it means the value was actually
read from the Registry.
__int64 def
|
The value to be used if the key is not found in
the Registry. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_BINARY |
ERROR_INVALID_DATA
|
The size of the Registry value is not
sizeof(__int64) |
ERROR_FILE_NOT_FOUND
|
The value was not found in the Registry, but
the def value was set. Note that load has returned
TRUE. |
BOOL RegistryInt64::store();
|
Stores the value to the Registry under the key specified for the variable.
The current contents of the .value member is what will be stored. If it
returns FALSE, use ::GetLastError to determine why it failed.
This is the .value member of the class.
RegistryString::RegistryString(UINT id, HKEY r = HKEY_CURRENT_USER)
|
Constructs a variable of type RegistryString.
Parmeters:
UINT id
|
The String Table ID of the Registry key under
which the value will be stored or from which the value will be retrieved. |
HKEY r
|
The root key under which the key is specified.
It will be found under the key
r\%IDS_PROGRAM_ROOT%\%id%
|
BOOL RegistryString::load(LPCTSTR def = NULL);
|
Loads a value from the Registry. Provides for a default value. The .value
member of the variable, a CString, will have either the value from the
Registry or the default value when this method returns. If the string parameter
value is NULL, the .value member is set to the empty string. If it
returns FALSE, use ::GetLastError to determine why it failed. If
the value was not found in the Registry and the default value is used, the value
returned is TRUE, and ::GetLastError will return
ERROR_FILE_NOT_FOUND. If the value was found, the value returned is TRUE
and ::GetLastError will return ERROR_SUCCESS.
int def
|
The value to be used if the key is not found in
the Registry. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_SZ,
REG_EXPAND_SZ, or REG_MULTI_SZ |
ERROR_FILE_NOT_FOUND
|
Returned FALSE: The error from
the Registry was ERROR_FILE_NOT_FOUND and the def
parameter was NULL. The value member is unchanged. Returned
TRUE: The error from the Registry was ERROR_FILE_NOT_FOUND but
the def parameter was non-NULL. The value member
has been set to the value of the def parameter. |
BOOL RegistryString::store();
|
Stores the value to the Registry under the key specified for the variable.
The current contents of the .value member is what will be stored. If it
returns FALSE, use ::GetLastError to determine why it failed.
This is the .value member of the class.
RegistryGUID::RegistryGUID(UINT id, HKEY r = HKEY_CURRENT_USER)
|
Constructs a variable of type RegistryGUID The Registry value is stored as a REG_BINARY.
Parmeters:
UINT id
|
The String Table ID of the Registry key under
which the value will be stored or from which the value will be retrieved. |
HKEY r
|
The root key under which the key is specified.
It will be found under the key
r\%IDS_PROGRAM_ROOT%\%id%
|
BOOL RegistryGUID::load(GUID * def = NULL);
|
Loads a value from the Registry. Provides for a default value. The .value
member of the variable will have either the value from the Registry or the
default value when this method returns. If it returns FALSE, use
::GetLastError to determine why it failed. If the value in the Registry is
not REG_BINARY the operation will fail with ERROR_INVALID_DATATYPE.
If the length of the value in the Registry is not sizeof(GUID) the operation will fail
with ERROR_INVALID_DATA. If the value is not found in the Registry, and
def is not NULL, the return type will be TRUE and
the value will be set to the contents of the referenced GUID. In this case,
::GetLastError will return ERROR_FILE_NOT_FOUND. If the default is
NULL, and the key is not found, the return value will be FALSE and the
value member is not changed; If the
return type is TRUE and ::GetLastError returns ERROR_SUCCESS
it means the value was actually read from the Registry.
GUID * def
|
The value to be used if the key is not found in
the Registry. If this is NULL, no default will be used and if the
value is not found in the Registry, the result will be FALSE and
::GetLastError will return ERROR_FILE_NOT_FOUND. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_BINARY |
ERROR_INVALID_DATA
|
Registry data size is not sizeof(GUID) |
ERROR_FILE_NOT_FOUND
|
Returned FALSE: The error from
the Registry was ERROR_FILE_NOT_FOUND and the def
parameter was NULL. The value member is unchanged. Returned
TRUE: The error from the Registry was ERROR_FILE_NOT_FOUND but
the def parameter was non-NULL. The value member
has been set to the value of the def parameter. |
BOOL RegistryGUID::store();
|
Stores the value to the Registry under the key specified for the variable.
The current contents of the .value member is what will be stored. If it
returns FALSE, use ::GetLastError to determine why it failed.
This is the .value member of the class.
RegistryFont::RegistryFont(UINT id, HKEY r = HKEY_CURRENT_USER)
|
Constructs a variable of type RegistryFont. The Registry value is stored as a
set of values under the registry key.
Parmeters:
UINT id
|
The String Table ID of the Registry key under
which the value will be stored or from which the value will be retrieved. |
HKEY r
|
The root key under which the key is specified.
It will be found under the key
r\%IDS_PROGRAM_ROOT%\%id%
|
|
|
Remarks:
Not all of the LOGFONT members are stored; only those indicated in the
table below are recorded. In the table the value %r% is symbolic for the
HKEY parameter of the constructor; the value %IDS_ROOT% is
whatever the string value of the IDS_ROOT string is in the STRINGTABLE;
and the value %id% represents the ID value obtained from the
STRINGTABLE based on the id parameter to the constructor.
LOGFONT member |
Registry type |
Registry Key |
Meaning |
lfHeight |
REG_DWORD |
%r%\%IDS_PROGRAM_ROOT%\%id%\lfHeight
|
The font height |
lfWidth |
not saved |
|
|
lfEscapement |
not saved |
|
|
lfOrientation |
not saved |
|
|
lfWeight |
REG_DWORD |
%r%\%IDS_PROGRAM_ROOT%\%id%\lfWeight
|
The font weight |
lfItalic |
REG_DWORD |
%r%\%IDS_PROGRAM_ROOT%\%id%\lfItalic
|
TRUE if italic, FALSE if not italic |
lfUnderline |
REG_DWORD |
%r%\%IDS_PROGRAM_ROOT%\%id%\lfUnderline
|
TRUE if underlined, FALSE if not |
lfStrikeout |
REG_DWORD |
%r%\%IDS_PROGRAM_ROOT%\%id%\lfStrikeout
|
TRUE if strkeout, FALSE if not |
lfCharSet |
not saved |
|
|
lfOutPrecision |
not saved |
|
|
lfClipPrecision |
not saved |
|
|
lfQuality |
not saved |
|
|
lfPitchAndFamily |
not saved |
|
|
lfFaceName |
REG_SZ |
%r%\%IDS_PROGRAM_ROOT%\%id%\lfFaceName
|
The face name |
BOOL RegistryFont::load(LOGFONT * def = NULL);
|
Loads a value from the Registry. Provides for a default value. The .value
member of the variable will have either the value from the Registry or the
default value when this method returns. If it returns FALSE, use
::GetLastError to determine why it failed. If the value in the Registry is
not REG_DWORD for integer values or REG_SZ for the face name, the operation will fail with ERROR_INVALID_DATATYPE.
If any integer value is not sizeof(DWORD) or the lfFaceName is
longer than LF_FACESIZE then the operation will fail
with ERROR_INVALID_DATA.
If the value is not found in the Registry, and
def is not NULL, the return type will be TRUE and
the value will be set to the contents of the referenced LOGFONT. In this case,
::GetLastError will return ERROR_FILE_NOT_FOUND. If the default is
NULL, and the key is not found, the return value will be FALSE and the
value member is not changed; If the
return type is TRUE and ::GetLastError returns ERROR_SUCCESS
it means the value was actually read from the Registry.
GUID * def
|
The value to be used if the key is not found in
the Registry. If this is NULL, no default will be used and if the
value is not found in the Registry, the result will be FALSE and
::GetLastError will return ERROR_FILE_NOT_FOUND. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type of one of the values stored in
the Registry was not appropriate. |
ERROR_INVALID_DATA
|
The length of the lfFaceName value is
greater than LF_FACESIZE. |
ERROR_FILE_NOT_FOUND
|
Returned FALSE: The error from
the Registry was ERROR_FILE_NOT_FOUND and the def
parameter was NULL. The value member is unchanged. Returned
TRUE: The error from the Registry was ERROR_FILE_NOT_FOUND but
the def parameter was non-NULL. The value member
has been set to the value of the def parameter. |
|
|
BOOL RegistryFont::store();
|
Stores the value to the Registry under the key specified for the variable.
The current contents of the .value member is what will be stored. If it
returns FALSE, use ::GetLastError to determine why it failed.
This is the .value member of the class.
RegistryWindowPlacement::RegistryWindowPlacement(UINT id, HKEY r = HKEY_CURRENT_USER)
|
Constructs a variable of type RegistryWindowPlacement. This will save any
32-bit signed or unsigned value. Shorter values should be saved as this type.
The Registry value is stored as a subtree of the registry key.
Parmeters:
UINT id
|
The String Table ID of the Registry key under
which the value will be stored or from which the value will be retrieved. |
HKEY r
|
The root key under which the key is specified.
It will be found under the key
r\%IDS_PROGRAM_ROOT%\%id%
|
Remarks:
The WINDOWPLACEMENT is stored as a set of values and subkeys of the
specified key of the constructor. In the table the value %r% is symbolic
for the HKEY parameter of the constructor; the value %IDS_ROOT% is
whatever the string value of the IDS_ROOT string is in the STRINGTABLE;
and the value %id% represents the ID value obtained from the
STRINGTABLE based on the id parameter to the constructor.
BOOL RegistryWindowPlacement::load(WINDOWPLACEMENT * def = NULL);
|
Loads a value from the Registry. Provides for a default value. The .value
member of the variable will have either the value from the Registry or the
default value when this method returns. If it returns FALSE, use
::GetLastError to determine why it failed. If the values in the Registry are
not REG_DWORD the operation will fail with ERROR_INVALID_DATATYPE. If the value is not found in the Registry, and
def is not NULL, the return type will be TRUE and
the value will be set to the contents of the referenced WINDOWPLACEMENT. In this case,
::GetLastError will return ERROR_FILE_NOT_FOUND. If the default is
NULL, and the key is not found, the return value will be FALSE and the
value member is not changed; If the
return type is TRUE and ::GetLastError returns ERROR_SUCCESS
it means the value was actually read from the Registry.
WINDOWPLACEMENT * def
|
The value to be used if the key is not found in
the Registry. If this is NULL, no default will be used and if the
value is not found in the Registry, the result will be FALSE and
::GetLastError will return ERROR_FILE_NOT_FOUND. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type of one of the values is not
REG_DWORD |
ERROR_FILE_NOT_FOUND
|
Returned FALSE: The error from
the Registry was ERROR_FILE_NOT_FOUND and the def
parameter was NULL. The value member is unchanged. Returned
TRUE: The error from the Registry was ERROR_FILE_NOT_FOUND but
the def parameter was non-NULL. The value member
has been set to the value of the def parameter. |
|
|
BOOL RegistryWindowPlacement::store();
|
Stores the value to the Registry under the key specified for the variable.
The current contents of the .value member is what will be stored. If it
returns FALSE, use ::GetLastError to determine why it failed.
This is the .value member of the class.
This uses a single key stored as REG_BINARY to hold the contents of a
CDWordArray. See also RegistryByteArray.
RegistryDWordArray::RegistryDWordArray(UINT id, HKEY r = HKEY_CURRENT_USER)
|
Constructs a variable of type RegistryDWordArray The Registry value is stored as a REG_BINARY.
Parmeters:
UINT id
|
The String Table ID of the Registry key under
which the value will be stored or from which the value will be retrieved. |
HKEY r
|
The root key under which the key is specified.
It will be found under the key
r\%IDS_PROGRAM_ROOT%\%id%
|
|
|
BOOL RegistryDWordArray::load();
|
Loads a value from the Registry. Provides for a default value. Note there is
no explicit default value; if the key is not found, an empy value array
results. The .value
member of the variable will have the value from the Registry, interpreted as an
array of DWORD values, when this method returns. If it returns FALSE, use
::GetLastError to determine why it failed. If the value in the Registry is
not REG_BINARY the operation will fail with ERROR_INVALID_DATATYPE.
If the length of the value in the Registry is not an integer multiple of
sizeof(DWORD) the operation will fail
with ERROR_INVALID_DATA. If the value is not found in the Registry, and
def is not NULL, the return type will be TRUE and
the value will be set to the contents of the referenced GUID. In this case,
::GetLastError will return ERROR_FILE_NOT_FOUND.
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_BINARY. |
ERROR_INVALID_DATA
|
The size of the data in the Registry is not an
integer multiple of sizeof(DWORD). |
ERROR_FILE_NOT_FOUND
|
Returned FALSE: The error from
the Registry was ERROR_FILE_NOT_FOUND and the size of the array is
set to 0. |
BOOL RegistryDWordArray::store();
|
Stores the value to the Registry under the key specified for the variable.
The current contents of the .value member is what will be stored. If it
returns FALSE, use ::GetLastError to determine why it failed.
This is the .value member of the class.
The Registry.cpp module provides for more
direct access to the Registry. All operations are performed relative to the
string specified by the String Table entry IDS_PROGRAM_ROOT.
These functions are used when you need closer control, for example, in
creating non-constant Registry keys that are computed on the fly.
To simplify the notation, I've adapted the notation for expansion of
environment strings. In this case, I use %name% to indicate the
expansion of the string identified in the String Table by the value name.
Thus %IDS_PROGRAM_ROOT% should be replaced, in the descriptions that
follow, by the string that IDS_PROGRAM_ROOTrepresents, and if the name
defines a variable or parameter in the program, by the string which is retrieved
by the integer represented by that variable or parameter.
In all cases, if the function returns FALSE, the error can found by
calling ::GetLastError(). In addition to the standard errors that can be
returned from the Registry API calls, if the value is the wrong type for the
operation, or of the wrong length, ::GetLastErro() will return ERROR_INVALID_DATA.
The default value is always present for scalar values, and optional for other
types of values. When a default value is provided, the operation will always
succeed and return TRUE even if the key is not found in the Registry.
However, if TRUE is returned and ::GetLastError returns
ERROR_FILE_NOT_FOUND, it means the key was not found and the default value
was used. If it returns TRUE and the key was found, ::GetLastError
will return ERROR_SUCCESS. If the default value is optional (usually
indicated by a NULL pointer to a value), the operation will always
return FALSE if the default is NULL and the key is not found in
the Registry.
In addition to the normal Registry errors that can be returned by
::GetLastError, the library will return ERROR_INVALID_DATA_TYPE or
ERROR_INVALID_DATA if the Registry type is incorrect or the data does not
meet certain size criteria.
If you use this header file, or if you use the RegVars.cpp
module, you must either include this in your compilation or link with the
compiled RegVars.obj file.
BOOL GetRegistryString(HKEY root, const CString & var, CString & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
CString & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be of type REG_SZ, will be
found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_SZ,
REG_EXPAND_SZ, or REG_MULTI_SZ |
BOOL SetRegistryString(HKEY root, const CString & var, const CString & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
const CString & val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a value of type REG_SZ. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_SZ,
REG_EXPAND_SZ, or REG_MULTI_SZ |
BOOL GetRegistryString(HKEY root, const CString & path, UINT var, CString & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\%var%
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
UINT var
|
The variable path that defines the variable,
represented by a String Table entry. If the path does not exist, the call
returns FALSE. Note that this string can include \ characters
describing more complex paths. |
CString & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be of type REG_SZ, will be
found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_SZ,
REG_EXPAND_SZ, or REG_MULTI_SZ |
BOOL SetRegistryString(HKEY root, const CString & path, UINT var, const CString & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\%var%
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
UINT var
|
The variable path that defines the variable,
contained in the String Table entry designated by the integer var.
If the path does not exist, it will be created. If there is any error
during the creation, this call returns FALSE. Note that this string
can include \ characters describing more complex paths. |
const CString & val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a value of type REG_SZ. |
BOOL GetRegistryString(HKEY root, const CString & path, const CString & var, CString & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\var
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
CString & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be of type REG_SZ, will be
found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_SZ,
REG_EXPAND_SZ, or REG_MULTI_SZ |
BOOL SetRegistryString(HKEY root, const CString & path, const CString & var, const CString & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\var
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
const CString & val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a value of type REG_SZ. |
BOOL GetRegistryInt(HKEY root, const CString & var, DWORD & val);
BOOL GetRegistryInt(HKEY root, const CString & var, int & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
DWORD & val
int & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be of type REG_DWORD, will be
found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_DWORD |
BOOL SetRegistryInt(HKEY root, const CString & var, DWORD val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
DWORD val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a value of type REG_DWORD. |
BOOL GetRegistryInt(HKEY root, const CString & path, UINT var, DWORD & val);
BOOL GetRegistryInt(HKEY root, const CString & path, UINT var, int & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\%var%
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
UINT var
|
The variable path that defines the variable,
represented by a String Table entry. If the path does not exist, the call
returns FALSE. Note that this string can include \ characters
describing more complex paths. |
DWORD & val
int & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be of type REG_DWORD, will be
found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_DWORD |
BOOL SetRegistryInt(HKEY root, const CString & path, UINT var, DWORD val);
BOOL SetRegistryInt(HKEY root, const CString & path, UINT var, int val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\%var%
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
UINT var
|
The variable path that defines the variable,
contained in the String Table entry designated by the integer var.
If the path does not exist, it will be created. If there is any error
during the creation, this call returns FALSE. Note that this string
can include \ characters describing more complex paths. |
DWORD val
int val
|
If the result of the call is TRUE, the
contents of this parameter has been stored as a value of type REG_DWORD. |
BOOL GetRegistryInt(HKEY root, const CString & path, const CString & var, DWORD & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\var
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
DWORD & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be of type REG_DWORD, will be
found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_DWORD |
BOOL SetRegistryInt(HKEY root, const CString & path, const CString & var, DWORD val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\path\var
|
const CString & path
|
The path from the root and program root to the
value, where the var parameter specifies the remainder of
the path. |
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
DWORD val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a value of type REG_DWORD. |
BOOL GetRegistryInt64(HKEY root, const CString & var, __int64 & val);
BOOL GetRegistryInt64(HKEY root, const CString & var, unsigned __int64 & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
__int64 & val
unsigned __int64 & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be an 8-byte value of type REG_BINARY,
will be found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_BINARY |
ERROR_INVALID_DATA
|
Length is not sizeof(__int64) |
BOOL SetRegistryInt64(HKEY root, const CString & var, __int64 val);
BOOL SetRegistryInt64(HKEY root, const CString & var, unsigned __int64 val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
__int64 val
unsigned __int64 val
|
If the result of the call is TRUE, the
contents of this parameter have been stored as an 8-byte value of type
REG_BINARY. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_BINARY |
ERROR_INVALID_DATA
|
Length is not 8 |
BOOL GetRegistryGUID(HKEY root, const CString & var, GUID & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
GUID & val
|
If the result of the call is TRUE, the
contents of the Registry, which must be a 16-byte value of type REG_BINARY,
will be found in this variable. If the result is FALSE it remains
unchanged. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_BINARY |
ERROR_INVALID_DATA
|
Length is not sizeof(GUID) |
|
|
BOOL SetRegistryGUID(HKEY root, const CString & var, const GUID & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
GUID val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as an 8-byte value of type
REG_BINARY. |
BOOL GetRegistryFont(HKEY root, const CString & var, LPLOGFONT val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
LPLOGFONT val
|
This structure will first be completely zeroed
out. If the result of the call is TRUE, the complete contents of
the Registry information for the font, as represented by the values under
the subkey var, will be found in this structure. If the
result is FALSE the fields will be partially filled in. The
correctness of the structure is not guaranteed. |
BOOL SetRegistryFont(HKEY root, const CString & var, const LOGFONT * val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
const LOGFONT * val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a set of values under the
subkey var. |
For a font, the var represents not a value, but a key under
which the values are stored. The following components of the LOGFONT
structure are stored. Note that this is not the complete set of possible values.
For example, I had no need to store font rotation information.
key |
type |
meaning |
lfHeight |
REG_DWORD
|
Font height |
lfWeight |
REG_DWORD
|
Font weight, FW_ symbols |
lfItalic |
REG_WORD
|
TRUE for italic font |
lfUnderline |
REG_DWORD
|
TRUE for underlined font |
lfStrikeout |
REG_DWORD
|
TRUE for strikeout font |
lfFaceName |
REG_SZ
|
Name of font |
In the example below, certain keys representing proprietary information have
been blanked out. What you see here are two keys, one for font and one for
window placement. The Font resource is shown expanded. Note that I do not
save all of the LOGFONT structure because I only needed the subset shown;
if you need more, the generalization should be obvious. The WindowPlacement
entry simply stores the coordinates of a WindowPlacement structure.
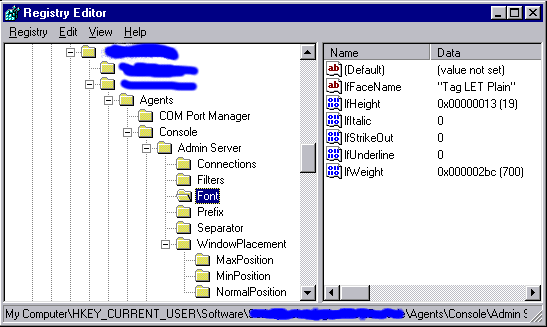
BOOL GetRegistryWindowPlacement(HKEY root, const CString & var, WINDOWPLACEMENT & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
WINDOWPLACEMENT & val
|
This structure will first be completely zeroed
out. If the result of the call is TRUE, the complete contents of
the Registry information for the WINDOWPLACEMENT, as represented by
the values under the subkey var, will be found in this
structure. If the result is FALSE the fields will be partially
filled in. |
BOOL SetRegistryWindowPlacement(HKEY root, const CString & var, const WINDOWPLACEMENT & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
const WINDOWPLACEMENT & val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a set of values under the
subkey var. |
For a font, the var represents not a value, but a key under
which the values are stored.
BOOL GetRegistryDWordArray(HKEY root, const CString & var, CDWordArray & result);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the variable. If
the path does not exist, the call returns FALSE. Note that this
string can include \ characters describing more complex paths. |
CDWordArray & result |
If successful, the values are placed in the CDWordArray
object. If the value is not found in the Registry, or there is some other
error, the result value is set to have a size of 0. |
::GetLastError()
|
Normal error responses from ::RegOpenKey
and ::RegQueryValueEx, plus the following |
ERROR_INVALID_DATA_TYPE
|
Registry type is not REG_BINARY |
ERROR_INVALID_DATA
|
Length is not not an integer multiple of
sizeof(DWORD). |
BOOL SetRegistryDWordArray(HKEY root, const CString & var, const CDWordArray & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The path that defines the variable. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
CDWordArray & val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a REG_BINARY value.. |
BOOL SetRegistryDWordArray(HKEY root, UINT var, const CDWordArray & val);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
UINT var
|
The path that defines the variable, expressed
as a STRINGTABLE entry. If
the path does not exist, it will be created. If there is any error during
the creation, this call returns FALSE. Note that this string can
include \ characters describing more complex paths. |
CDWordArray & val
|
If the result of the call is TRUE, the
contents of this parameter will be stored as a REG_BINARY value.. |
Note that this can be invoked also by using the remove member of the
RegistryVar superclass.
BOOL DeleteRegistryKey(HKEY root, const CString & keyname);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & keyname
|
The variable path that defines the key. If the
path does not exist, the call returns TRUE, but ::GetLastError
will then indicate ERROR_FILE_NOT_FOUND. . If the path exists, an
attempt is made to delete it; if it is successfully deleted, the return
value is TRUE and ::GetLastError will return
ERROR_SUCCESS. If there is a deletion failure, it returns FALSE. |
BOOL DeleteRegistryValue(HKEY root, const CString & var);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\var
|
const CString & var
|
The variable path that defines the key. If the
path does not exist, the call returns TRUE, but ::GetLastError
will then indicate ERROR_FILE_NOT_FOUND. . If the path exists, an
attempt is made to delete it; if it is successfully deleted, the return
value is TRUE and ::GetLastError will return
ERROR_SUCCESS. If there is a deletion failure, it returns FALSE. |
BOOL GetRegistryKey(HKEY root, const CString & keyname, HKEY & key, DWORD access = KEY_ALL_ACCESS);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The variable path that defines the parent key.
If the path does not exist, an attempt is made to create it. If the
creation succeeds, or the path already exists, the key value
is set, and the call returns TRUE. If the path does not exist, and
cannot be created, the call returns FALSE. |
HKEY & key
|
The Registry key handle which represents the
key defined by the keyname path for the current program
root. This value is valid only if the function returns TRUE. |
DWORD access |
The desired access with which the key is
opened. If not specified, KEY_ALL_ACCESS is used. |
BOOL FindRegistryKey(HKEY root, const CString & keyname, HKEY & key, DWORD access = KEY_ALL_ACCESS);
|
HKEY root
|
The root relative to which the key is found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The variable path that defines the key. If the
path does not exist, the return value is FALSE and no attempt is
made to create it. If the path exists, a key handle is opened and returned
in the key provided. Note that this string can include \
characters describing more complex paths. |
HKEY & key
|
The Registry key handle which represents the
key defined by the keyname path for the current program
root. This value is valid only if the function returns TRUE. |
DWORD access |
The desired access with which the key is
opened. If not specified, KEY_ALL_ACCESS is used. |
BOOL EnumRegistryKeys(HKEY root, const CString & keyname, CStringArray & keys);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The variable path that defines the parent key.
If the path does not exist, the call returns FALSE. If the path
exists, the subkeys are enumerated and returned in the result CStringArray. |
CStringArray & result |
An array which contains the keys which are
found. If no keys are found, this is set to be empty. If there is an error,
there may be a partially-filled-in array but its correctness is not defined. |
BOOL EnumRegistryKeys(HKEY root, UINT keyname, CStringArray & keys);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\%keyname%
|
UINT keyname
|
The variable path that defines the parent key.
If the path does not exist, the call returns FALSE. If the path
exists, the subkeys are enumerated and returned in the result CStringArray. |
CStringArray & result |
An array which contains the keys which are
found. If no keys are found, this is set to be empty. If there is an error,
there may be a partially-filled-in array but its correctness is not defined. |
BOOL EnumRegistryValues(HKEY root, const CString & keyname, CStringArray & keys);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The variable path that defines the parent key.
If the path does not exist, the return value is FALSE. If the path
exists, the names of all the values under the key are returned in the
result CStringArray. Note that the keyname string can
include \ characters describing more complex paths. |
CStringArray & result |
An array which contains the keys which are
found. If no keys are found, this is set to be empty. If there is an error,
there may be a partially-filled-in array but its correctness is not defined. |
[Set/Get]RegistryValues
The idea of this is to have a CStringArray or CDWordArray of
values which will be stored as individual values in the Registry. The values
will be stored as a sequence of names 00000, 00001, 00002,
and so on. This can conveniently be used to store sequences of string or
DWORD values.
BOOL SetRegistryValues(HKEY root, const CString & keyname, const CStringArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The path that defines the parent key. If the
path does not exist, it will be created. If the path
exists, the names of all the values under the key are deleted, then
replaced by the values in the
CStringArray. Note that the keyname string can
include \ characters describing more complex paths. |
const CStringArray & values |
An array which contains the values to be
stored. |
BOOL SetRegistryValues(HKEY root, UINT keyid, const CStringArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
UINT keyid
|
The STRINGTABLE id that defines the
parent key. If the path does not exist, it will be created. If the path
exists, the names of all the values under the key are deleted, then
replaced by the values in the
CStringArray. Note that the keyname string can
include \ characters describing more complex paths. |
const CStringArray & values |
An array which contains the values to be
stored. |
BOOL SetRegistryValues(HKEY root, const CString & keyname, const DWordArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The path that defines the parent key. If the
path does not exist, it will be created. If the path
exists, the names of all the values under the key are deleted, then
replaced by the values in the
CDWordArray. Note that the keyname string can
include \ characters describing more complex paths. |
const CStringArray & result |
An array which contains the values to be
stored. |
BOOL SetRegistryValues(HKEY root, UINT keyid, const CDWordArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
UINT keyid
|
The STRINGTABLE id that defines the
parent key. If the path does not exist, it will be created. If the path
exists, the names of all the values under the key are deleted, then
replaced by the values in the
CDWordArray. Note that the keyname string can
include \ characters describing more complex paths. |
const CDWordArray & values |
An array which contains the values to be
stored. |
BOOL GetRegistryValues(HKEY root, const CString & keyname, CStringArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The path that defines the parent key. If the
path does not exist, the result will be FALSE and the size of
values will be set to 0. If the path
exists, the names of all the values under the key are enumerated, and
their values placed in the
CStringArray. Note that the keyname string can
include \ characters describing more complex paths. |
CStringArray & values |
An array which contains the values read from
the Registry. |
BOOL GetRegistryValues(HKEY root, UINT keyid, CStringArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
UINT keyid
|
The STRINGTABLE id that defines the
parent key. If the path does not exist, the result will be FALSE and
the size of values will be set to 0. If the path
exists, the names of all the values under the key are enumerated, and
their values placed in the
CStringArray. Note that the keyname string can
include \ characters describing more complex paths. |
CStringArray & values |
An array which contains the values read from
the Registry. |
BOOL GetRegistryValues(HKEY root, const CString & keyname, DWordArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
const CString & keyname
|
The path that defines the parent key. The path
that defines the parent key. If the path does not exist, the result will be
FALSE and the size of values will be set to 0. If the path
exists, the names of all the values under the key are enumerated, and
their values placed in the
CDWordArray. Note that the keyname string can
include \ characters describing more complex paths. |
const CStringArray & result |
An array which contains the values read from
the Registry. |
BOOL SetRegistryValues(HKEY root, UINT keyid, CDWordArray & values);
|
HKEY root
|
The root relative to which the keys are found,
typically HKEY_CURRENT_USER or HKEY_LOCAL_MACHINE. The key
will be evaluated as
root\%IDS_PROGRAM_ROOT%\keyname
|
UINT keyid
|
The STRINGTABLE id that defines the
parent key. If the path does not exist, the result will be FALSE and
the size of values will be set to 0. If the path
exists, the names of all the values under the key are enumerated, and
their values placed in the
CDWordArray. Note that the keyname string can
include \ characters describing more complex paths. |
const CDWordArray & values |
An array which contains the values read from
the Registry. |
This function is used to obtain the key path given a string name or
STRINGTABLE id
CString GetKeyPath(const CString & key)
CString GetKeyPath(UINT keyid)
|
const CString & key
|
The string representing a key name. The value
returned will be%IDS_PROGRAM_ROOT%\key
|
UINT keyid
|
The string representing the key name. The value
returned will be%IDS_PROGRAM_ROOT%\%keyid%
|
CreateKey creates an actual key entry in the Registry. If an absolute
key name is provided, that key is created. If a relative key name is provided,
it is created under the IDS_PROGRAM_ROOT
value. The key is created in the Registry and then closed.
BOOL CreateKey(HKEY root, const CString & key, DWORD access = KEY_ALL_ACCESS)
|
HKEY root
|
The root key. HKEY_CURRENT_USER is the
most common value used here |
const CString & key
|
The string representing a key name. The key
created will beroot\%IDS_PROGRAM_ROOT%\key
|
DWORD access
|
The desired access to the key |
OpenKey opens a key and returns the HKEY value. This is
to allow explicit operations to be performed. If an absolute key name is
provided, that key is opened. If a relative key name is provided, it is created
under the IDS_PROGRAM_ROOT value. The
return value is the HKEY, or NULL if an error occured
HKEY OpenKey(HKEY root, const CString & key, DWORD access = KEY_ALL_ACCESS)
|
HKEY root
|
The root key. HKEY_CURRENT_USER is the
most common value used here |
const CString & key
|
The string representing a key name. The key
opened will beroot\%IDS_PROGRAM_ROOT%\key
|
DWORD access
|
The desired access to the key. |
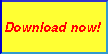
Restrospective
(2001) There are probably some things I would do differently today (I wrote most of
this code in 1996), such as passing in references to arrays instead of returning
pointers to created arrays, but this is the code as it stands today.
(2005) I have made significant upgrades. New classes for
RegistryDWordArray, RegistryGUID, RegistryInt64, and
RegistryWindowPlacement have been added. The enumeration functions now take
reference parameters, and the methods which formerly returned allocated arrays
now take array reference parameters and fill them in. The load and
store methods now return BOOL instead of void, and
consistently the substitution of the default value is indicated by returning a
success indicator with ::GetLastError returning ERROR_FILE_NOT_FOUND;
if the value is found and the default is not used, ::GetLastError will
return ERROR_SUCCESS. A new remove method has been added. I added
GetKeyPath so the program can handle other explicit Registry operations.
The views expressed in these essays are those of the
author, and in no way represent, nor are they endorsed by, Microsoft.
Send mail to newcomer@flounder.com
with questions or comments about this web site.
Copyright © 2001 The Joseph M. Newcomer Co.
All Rights Reserved.
Last modified:
May 14, 2011